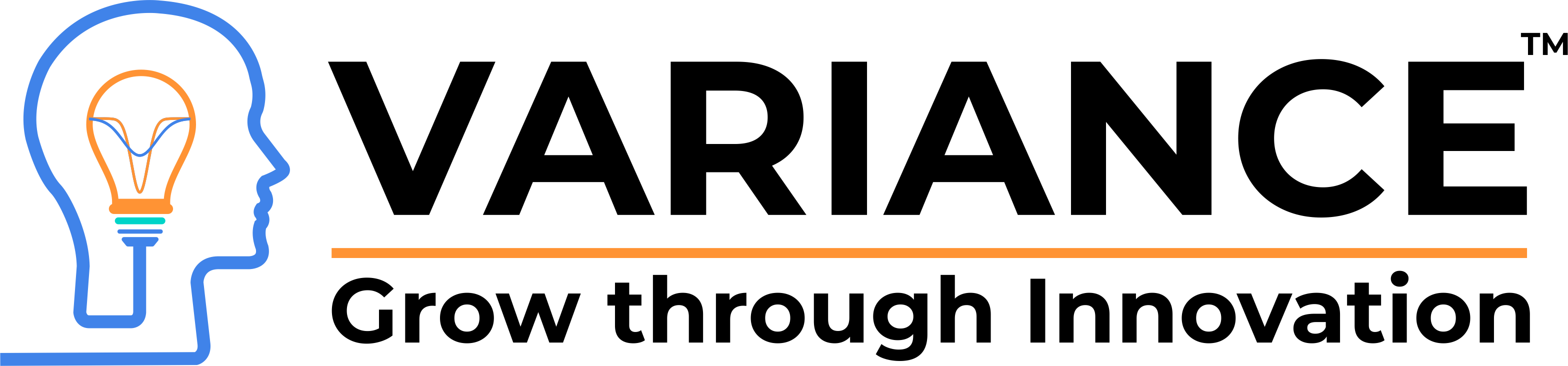
Pioneer in Offering CRM Solutions since 2010...
The client specializes in streamlined legal and lender process outsourcing. They are providing various services like Digital Mortgage Documentation and Settlements, Mortgagee in Possession Services, Mortgage Debt Recovery, Online Search Services, Legal Agent Services, etc.
Client already created a desktop application using Delphi, Web API 2 (to communicate with desktop application), Customer portal using .NET MVC Framework and SAP Sybase SQL as database. The Client has multiple small modules available which are interconnected and tightly coupled. Database connectivity is maintained using NHibernate ORM. There are separate servers for database, web application, utilities, file service, etc. Deployment process on each server was manual. Dependency injection is managed by Autofac.
The client is a well known entity in the Legal and lender process industry. It has it’s own various challenges:
We convinced the client to migrate the legacy application to modern .NET application with the latest .NET framework.
Ready to start your dream project?
The goal is to migrate a legacy desktop based application to .NET 7. Variance Infotech studied various technology options and decided to go with .NET 7, SQL Server as base technology. We used RabbitMQ and Action Step to implement a publish subscribe pattern.
The decision to migrate to .NET 7 was driven by the Legal Agency's aim to modernise their technology stack. .NET 7 provides advanced features, improved performance, and robust support, aligning with the agency's commitment to streamlined legal and lender process outsourcing.
The migration to .NET 7 enhances the capabilities of the Legal Agency in providing services such as Digital Mortgage Documentation and Settlements, Mortgagee in Possession Services, Mortgage Debt Recovery, Online Search Services, and Legal Agent Services. It enables a more efficient and modernised service delivery.
specific functionalities and features, including performance optimizations, new language features, and compatibility improvements. These elements contribute to a more robust and future-ready application for the Legal Agency.
The migration process is meticulously planned and executed, involving steps such as code refactoring, compatibility testing, and performance optimization. The goal is to ensure a smooth transition with minimal disruptions to the agency's legal and lender process outsourcing activities.
Yes, the migration process includes considerations for legal compliance to uphold the agency's commitment to regulatory requirements. This encompasses data security, privacy, and any legal standards relevant to the services provided by the agency.
Variance InfoTech Pvt Ltd.
608/609, 6th floor - Abhishree Adroit,
Vastrapur, Ahmedabad, 380015, India
Phone : +91-7016851729
Email : info@varianceinfotech.com
Variance InfoTech LLC
30 N Gould St. Sheridan,
WY 82801 USA
Phone : +16305340223
Email : info@varianceinfotech.com
©Copyright 2024. All Rights Reserved | Privacy Policy
We use cookies to provide better experience on our website. By continuing to use our site, you accept our Cookies and Privacy Policy.
Accept